@root thx bro
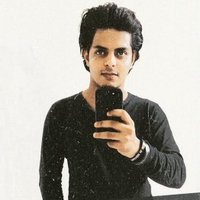
Posts made by b6
-
Top 7 Javascript Best Practices For Beginners 👍
1. Always use camelcase when declare variables.
❌ Don't Use
var first_name = document.ElementById("firstname").value; var date_of_birth = document.ElementById("dob").value;
✔ Use
var firstName = document.ElementById("firstname").value; var dateOfBirth = document.ElementById("dob").value;
2. Use shorten IF in small conditions.
❌ Don't Use
//Un-Compressed Code if ( age < 19 ) { isMajor = false; } else { isMajor = true; }
✔ Use
//Compressed Code isMajor = ( age < 19 ) ? false : true;
3. Declare Variables outside of loop.
Not in all cases. Do it when you use common components/functions/DOMs inside the loop.
❌ Don't Use
for(var i = 0; i < someArray.length; i++) { var container = document.getElementById('container'); container.innerHtml += 'my number: ' + i; } /* This will load the DOM each time of the loop. Will slowdown your application performance in big cases. */
✔ Use
var container = document.getElementById('container'); for(var i = 0; i < someArray.length; i++) { container.innerHtml += 'my number: ' + i; console.log(i); }
4. Reduce the single global variables.
❌ Don't Use
var userId = '123456'; var userName = 'username'; var dateOfBirth = '06-11-1998'; function doSomething(userId,userName,dateOfBirth){ //Your Implementation }
✔ Use
//Make a collection of data var userData = { userId : '123456', userName : 'username', dateOfBirth : '06-11-1998' } function doSomething(userData){ //Your Implementation } function doSomethingElse(userData.userId){ //Your Implementation }
5. Always comment your works & function input,outputs.
❌ Don't Use
function addNumbers(num1, num2){ return num1 + num2; } function getDataById(id){ return someDataArray[id]; }
✔ Use
/* * Adding two numbers * @para num1 (int), num2 (int) * @return int */ function addNumbers(num1, num2){ return num1 + num2; } /* * Adding two numbers * @para id (int) * @return Array */ function getDataById(id){ return someDataArray[id]; }
6. Always Build/Minify your JS before move it to live.
Commenting your works, New lines & other best practices will
increase
your javascript file size. We need this stuffs in Development Environment only.So Build & Minify your javascript file using build tools. It will compress your javascript to load much faster.
Asserts Build Tool : Webpack
Online Javascript Minifier : javascript-minifier
7. Load your scripts just before </body> Tags.
❌ Don't Use
<head> <!--Don't Load JS files here.--> </head>
<body> <!--Don't Load JS files here.-->
✔ Use
<!--Load JS files here.--> </body> </html>
-
RE: What are the best cpanel alternatives (free)?
@root The best way to handle different sources files and configurations depends on region / feature / client, Use build tools like Jenkins / Travis-CI, It will build correct .env and config for each deployments
-
⚖ Load Balancing vs ⚙ Failover
:scales: Load Balancing vs :hourglass: Failover
Apart from Application Development, To become a
DevOps Engineer
orSystem Admin
you need to learn some production leveldeployment
andmaintenance
technique to design betterserver architecture
to providehigh available service
.In this small blog, We will see about 2 important things,
- Load Balancing
- Failover
Both are
server setup techniques
to provideHigh Available
orZero Downtime
services.Let's See one by one.
The problem
When you deploy our application. The server will serve the service to our clients.
The client will send arequest
to server, Then our server willresponse
to client.When so many clients use our service / app, They will send
millions of requests per second
. Everything has a limited capacity. We can do limited tasks using our two hands.Like that Server will handle
only limited requests
per second (around 1000 req/ sec But it depends on the internal architecture).In above case, When server meet the maximum limit, It will get trouble to process the requests. So the
server will stop service / hang automatically
. This is what we calledServer Down
. In this case, Client's will not access our service / application / website which is hosted in that perticlular server.To avoid this problem, some server softwares automatically decline the clients' requests when reach limit. But this will create a
bad opinion
:angry: :angry: on ourusers'
orclients'
mind about our service. They will be disappointed when they couln't access our service.Here we need to implement
Load Balancing
orFailover
.
The Load Balancing
The name will give you a idea. Just think balance the load.
Load Balancing means
divide
anddistribute
thetraffic (requests)
between more than one servers.For example
You have 3 servers, Each server will handle 1000 requests per second.When you receive 2500 requests per second, The load balancer will divide the requests and serve to 3 servers something like,
Server 1 - 900 Req / S
Server 2 - 900 Req / S
Server 3 - 700 Req / SHere your servers will work together without getting stucked.
There are
2 types
ofload balancers
.- Physical Load Balancer
- Logical Load Balancer
Physical load Balancer
This is a device looks like a
network switch
.Connected with servers to balance incomming traffic.
Logical Load Balancer
Thit will run as a service inside the
main server
orproxy
. It will receive allrequests
then transfer to other services insame server
orsubservers
.Something like this,
Main Server - yourapp.com
The main server will get request, Then will forward & balance between below services.
App1 - localhost:80
App2 - localhost:8080
App3 - localhost:8000
App4 - localhost:8001The
Nginx
is a famousweb server
with built-inreverse proxy
&load balancer
Sample Load Balancer Configuration of Nginx.
http { upstream myapp1 { server srv1.example.com; server srv2.example.com; server srv3.example.com; } server { listen 80; location / { proxy_pass http://myapp1; } } }
Click Here to Read More About nginx Load Balancing.
Failover
Failover
is little different fromload balancing
. Here we are not going to balance the load.Here also we need more than 1 server. But we will fireup other server instead of one, When that server went down.
Confusing? For Example
We have following 2 servers.
Server 1 : 192.168.8.100
Server 2 : 192.168.8.103
Here, We don't use both servers at the same time. We will start the second server, When the first server went down.
When our first server
stop working
, Thefailover
machanismautomatically turn-on
server 2.
These are the main concepts of
Load Balancing
andFailover
. Hope now you have learn new thing.Just put a comment, If you want to write about anything else. Or if you have any questions.
Thank you!
With :hearts: B6.
-
🔑 Encryption vs 🔒 Hashing
:key: Encryption vs :shield: Hashing
Introduction
Encryption
andHashing
. The two main terms incryptogrphy
. We know both techniques are using to hide actual data in programming. But there are few major differences between.Let's see the very basic concept & difference between Encryption and Hashing.
Encryption
Encryption is the process of using an algorithm to transform information to make it unreadable for unauthorized users. - Techopedia
Encryption
is a kind of algorithm to transform the data to unreadable format or specific format that can't be read by anyone else.It looks like,
Before Encrypt - Hi, How are you?
After Encrypt - dahj963hqd92h2hr2f hf9vb3y9863b52c3569Only the
system
orspecific user
can decrypt the data.The lenth of encrypted data depends on the actual data lenth. If you input small data, the encrypted data also will be smaller. If you input huge data, the encrypted data will be larger.
The encryption machanism has two type of keys.
1. Public Key - Open key that use to encrypt data.
2. Private Key - Secret key that use to decrypt the encrypted data.
Hashing
Hashing
is also working likeencryption
. ButHash
doesn't have keys likepublic
&private
.And Also,
Hashing
's output doesn't depends on the input size. Its' output will be a fixed size of string.It means, imagine the hashing function
hash()
returns 32 characters output, The output will not be increased or decresed.echo hash('a') 0cc175b9c0f1b6a831c399e269772661 //32 chars output echo hash('Data encryption translates data into another form, or code, so that only people with access to a secret key (formally called a decryption key) or password can read it. Encrypted data is commonly referred to as ciphertext, while unencrypted data is called plaintext. Currently, encryption is one of the most popular and effective data security methods used by organizations. Two main types of data encryption exist - asymmetric encryption, also known as public-key encryption, and symmetric encryption') fc2a2d702e1af572e4fe4dddb31ccc90 //32 chars output
The another thing is, We can't reverse the hash. It means we
can
hash a data. But wecan't
get data again from hash.
Encryption Hashing Output length not Fixed Fixed Output length Can retrieve data again Can't retrieve data again Use as a trusted data Use as a trusted and unique identifier
When use Encryption and Hashing ?
Use cases of
hashing
,- Store Passwords in Database.
- Compare file hashes for check damage (Check Sum).
- Compare data for difference or changes.
Use case of
encryption
,- Store sensitive data.
- Transfer data over network / internet.
- Make dedicated file system / extensions.
Thank you!
With :hearts: B6.