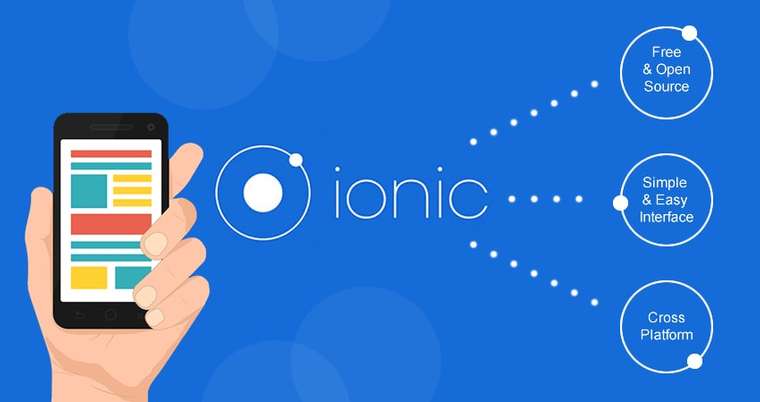
What is Ionic Framework
Ionic is a complete open-source SDK for hybrid mobile app development created by Max Lynch, Ben Sperry and Adam Bradley of Drifty Co. in 2013. The original version was released in 2013 and built on top of AngularJS and Apache Cordova. The more recent releases, known as Ionic 3 or simply "Ionic", are built on Angular. Ionic provides tools and services for developing hybrid mobile apps using Web technologies like CSS, HTML5, and Sass. Apps can be built with these Web technologies and then distributed through native app stores to be installed on devices by leveraging Cordova.
Installing Ionic
Ionic apps are created and developed primarily through the Ionic command line utility (the “CLI”), and use Cordova to build/deploy as a native app. This means we need to install a few utilities to get developing.
Installing Node and NPM
The quickest way to get Node and NPM installed on your machine is through the NodeJS installer. After installing open a terminal and run npm --version
and node --version
to verify you have everything installed correctly.
Installing Ionic CLI
Run below command on your terminal
$ npm install -g ionic cordova
Note: The -g means this is a global install, so for Windows you will need to open an Admin command prompt. For Mac/Linux, you might need to run the command with sudo.
Creating Ionic App
To create a blank app run below command
ionic start helloWorld blank
start
will tell the CLI create a new app.helloWorld
is the App name.blank
is starter template
Along with the blank template, Ionic also provides the following official templates:
tabs
: a simple 3 tab layout
sidemenu
: a layout with a swipable menu on the side
blank
: a bare starter with a single page
super
: starter project with over 14 ready to use page designs
tutorial
: a guided starter project
If you don’t specify a template at the start, you will be prompted to pick one.
Running our App
To run your app, cd into the directory that was created.
$ cd helloWorld
$ ionic serve
In the next article i'll go over the project structure created by the ionic start
command
References